Core Concepts
Polymorphic Serialisation
WebSock contains a robust serialisation framework that enables automagic integration with Unreal's property system, removing the manual toil and error-prone nature of writing manual (de)serialisation code.
Overview
Taking advantage of UWebSockLib
is crucial to using WebSock to its full effect: its powerful USTRUCT <-> JSON (de)serialisation is easy to use in Blueprints and C++.
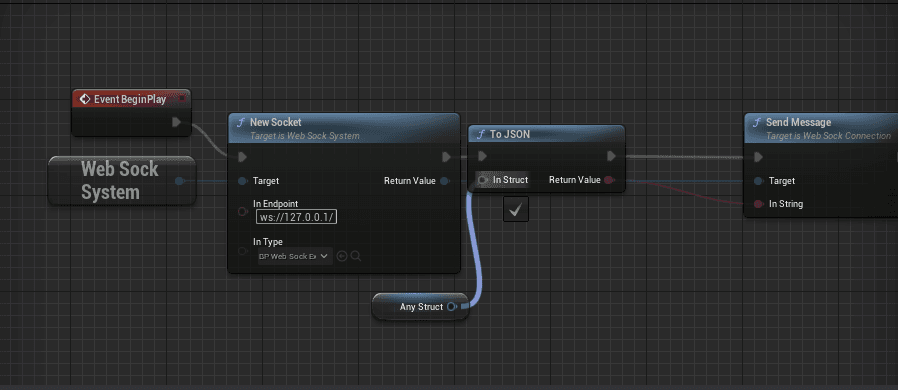
Example
WebSock enables the rapid construction of type-safe APIs using websockets.
USTRUCT(BlueprintType)
struct FMyStruct
{
UPROPERTY(BlueprintReadOnly, VisibleAnywhere)
FString Msg;
UPROPERTY(BlueprintReadOnly, VisibleAnywhere)
int32 Numba;
}
static void SendExampleNumba(){
auto WebSock = UWebSockSystem::Get(GetWorld());
if (auto Connection = WebSock->NewSocket("ws://127.0.0.1"))
{
Connection->Send<FMyStruct>({
"hello world",
42
});
}
}
The above example could be received in any popular language as a JSON payload:
{
"Msg": "hello world",
"Numba": 42
}
By reducing the moving parts in sending and receiving FMyStruct
we enable safe interoperability with external services and remove the cognitive burden of manually tracking multiple FMyStruct
definitions across a codebase.
Benefits
- We can keep
FMyStruct
in one place inside an Unreal project without writing wrappers or glue code to handle transport; - Avoid code duplication by using Blueprint wildcards, and C++20 template parameters;
- Lowers LOC and maintenance burden alongside providing UE native type-safety;
- Compact API is easy to implement in a variety of projects that require websocket networking.