Introduction
Getting started
Integrate WebSock into your Unreal project in seconds
Quick Start
Rapid onboarding with our step-by-step setup guide that's under 60 seconds
Core Concepts
Dive deeper on the high-level vision and low-level internals that power WebSock
Motivating Examples
Unleash the next-generation of websocket networking in UE
API Reference
Using WebSock with C++ and Blueprints accelerates development & reduces bugs
WebSock contains a robust serde framework that provides type-safe polymorphic serialisation within the Unreal property system, and an asynchronous runtime Game Instance encapsulated subsystem for managing websocket transport.
Quick Start
You can grab WebSock from: WebSock (Unreal Marketplace)
Config
Plugin
Inside Unreal Editor, open Plugins
and enable WebSock
for your project, you may have to restart your Editor to continue.
Project
Inside Project Settings
, the WebSock Settings
tab can be used to define endpoints and handlers that are managed entirely by WebSock.
Automatic lifetime management
Handlers defined in WebSock Settings are useful for long-lived connections to external services that are brought up early in the UEngine lifetime e.g. auth microservice, persistent data channels, etc.
Example
USTRUCT(BlueprintType)
struct FMyStruct
{
UPROPERTY()
FString Msg;
}
static void SendHelloWorld(UWebSockConnection* Connection){
if (Connection)
{
Connection->Send<FMyStruct>({"hello world"});
}
}
Sending any USTRUCT payload safely is easy in C++ and Blueprints.
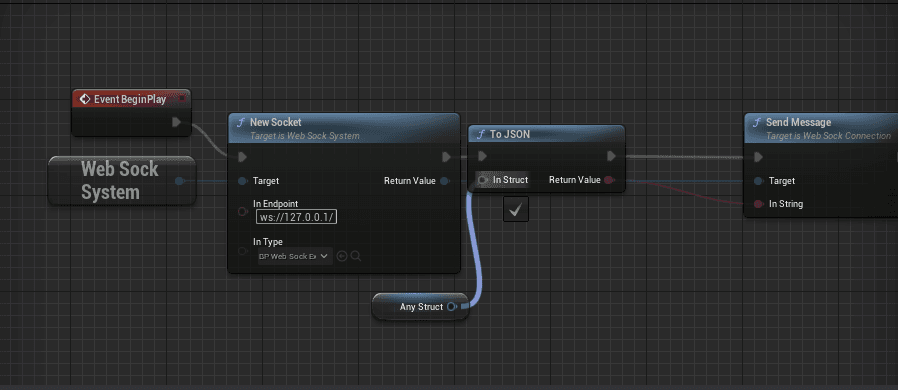
Further reading
More detailed information on sending, receiving and (de)serialisation can be found by reading the WebSock API reference and walkthrough examples
Overview
WebSock is event-driven by design, allowing for the rapid development of complex systems atop a handful of simple websocket events.
Your first WebSock
Subclassing UWebSockConnection
in either Blueprint or C++ allows you to build stateless, or stateful, handlers that implement key BlueprintNativeEvents
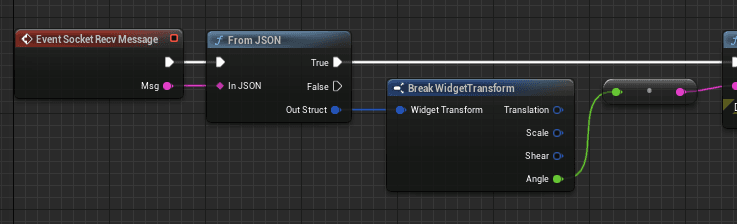
Architecture
WebSock is minimal by nature, and can be used to compose elegant architecture from only the following classes:
- UWebSockConnection, an event-driven endpoint handler;
- Subclassed by BP or C++, a UWebSockConnection connects to an endpoint to send and receive payloads inside Unreal
- UWebSockSystem, a Game Instance Subsystem;
- Automatically manages the lifetimes of UWebSockConnections
- Configuration is handled by UWebSockSettings inside Project Settings
- UWebSockLib, a Blueprint Function Library;
- Provides Blueprint-exposed polymorphic serialisation functionality